How to get second child in jquery
jQuery :nth-child() Selector
❮ jQuery Selectors
Example
Select each <p> element that is the third child of its parent:
$("p:nth-child(3)")
Try it Yourself »
Definition and Usage
The :nth-child(n) selector selects all elements that are the nth child, regardless of type, of their parent.
Tip: Use the :nth-of-type() selector to select all elements that are the nth child, of a particular type, of their parent.
Syntax
:nth-child(n|even|odd|formula)
Parameter | Description |
---|---|
n | The index of each child to match. Must be a number. The first element has the index number 1. |
even | Selects each even child element |
odd | Selects each odd child element |
formula | Specifies which child element(s) to be selected with a formula (an + b). Example: p:nth-child(3n+2) selects each 3rd paragraph, starting at the 2nd child element |
Try it Yourself - Example
Select each <p> element that is the second child of all <div> elements
How to select each <p> element that is the second child of all <div> elements.
Using a formula (an + b)
How to use a formula (an + b) to select different child elements.
Using "even" and "odd"
How to use even and odd to select different child elements.
Difference between :nth-child(), :nth-last-child(), :nth-of-type() and :nth-of-last-type()
The difference between p:nth-child(2), p:nth-last-child(2), p:nth-of-type(2) and p:nth-of-last-type(2).
❮ jQuery Selectors
COLOR PICKER
Top Tutorials
HTML TutorialCSS Tutorial
JavaScript Tutorial
How To Tutorial
SQL Tutorial
Python Tutorial
W3.CSS Tutorial
Bootstrap Tutorial
PHP Tutorial
Java Tutorial
C++ Tutorial
jQuery Tutorial
Top References
HTML ReferenceCSS Reference
JavaScript Reference
SQL Reference
Python Reference
W3.
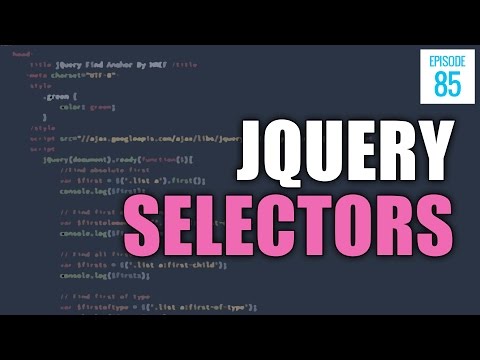
Bootstrap Reference
PHP Reference
HTML Colors
Java Reference
Angular Reference
jQuery Reference
Top Examples
HTML ExamplesCSS Examples
JavaScript Examples
How To Examples
SQL Examples
Python Examples
W3.CSS Examples
Bootstrap Examples
PHP Examples
Java Examples
XML Examples
jQuery Examples
FORUM | ABOUT
W3Schools is optimized for learning and training. Examples might be simplified to improve reading and learning. Tutorials, references, and examples are constantly reviewed to avoid errors, but we cannot warrant full correctness of all content. While using W3Schools, you agree to have read and accepted our terms of use, cookie and privacy policy.
Copyright 1999-2022 by Refsnes Data. All Rights Reserved.
W3Schools is Powered by W3.CSS.
:nth-last-child() - CSS: Cascading Style Sheets
The :nth-last-child()
CSS pseudo-class matches elements based on their position among a group of siblings, counting from the end.
The nth-last-child
pseudo-class is specified with a single argument, which represents the pattern for matching elements, counting from the end.
:nth-last-child( <nth> [ of <complex-selector-list> ]? )
Keyword values
odd
-
Represents elements whose numeric position in a series of siblings is odd: 1, 3, 5, etc., counting from the end.
even
-
Represents elements whose numeric position in a series of siblings is even: 2, 4, 6, etc., counting from the end.
Functional notation
<An+B>
-
Represents elements whose numeric position in a series of siblings matches the pattern
An+B
, for every positive integer or zero value ofn
, where:A
is an integer step size,B
is an integer offset,n
is all nonnegative integers, starting from 0.
It can be read as the
An+B
-th element of a list. The index of the first element, counting from the end, is1
. TheA
andB
must both have<integer>
values.
Example selectors
tr:nth-last-child(odd)
ortr:nth-last-child(2n+1)
-
Represents the odd rows of an HTML table: 1, 3, 5, etc., counting from the end.
tr:nth-last-child(even)
ortr:nth-last-child(2n)
-
Represents the even rows of an HTML table: 2, 4, 6, etc., counting from the end.
:nth-last-child(7)
-
Represents the seventh element, counting from the end.
:nth-last-child(5n)
-
Represents elements 5, 10, 15, etc., counting from the end.
:nth-last-child(3n+4)
-
Represents elements 4, 7, 10, 13, etc.
, counting from the end.
:nth-last-child(-n+3)
-
Represents the last three elements among a group of siblings.
p:nth-last-child(n)
orp:nth-last-child(n+1)
-
Represents every
<p>
element among a group of siblings. This is the same as a simplep
selector. (Sincen
starts at zero, while the last element begins at one,n
andn+1
will both select the same elements.) p:nth-last-child(1)
orp:nth-last-child(0n+1)
-
Represents every
<p>
that is the first element among a group of siblings, counting from the end. This is the same as the:last-child
selector.
Table example
HTML
<table> <tbody> <tr> <td>First line</td> </tr> <tr> <td>Second line</td> </tr> <tr> <td>Third line</td> </tr> <tr> <td>Fourth line</td> </tr> <tr> <td>Fifth line</td> </tr> </tbody> </table>
CSS
table { border: 1px solid blue; } /* Selects the last three elements */ tr:nth-last-child(-n + 3) { background-color: pink; } /* Selects every element starting from the second to last item */ tr:nth-last-child(n + 2) { color: blue; } /* Select only the last second element */ tr:nth-last-child(2) { font-weight: 600; }
Result
Quantity query
A quantity query styles elements depending on how many of them there are. In this example, list items turn red when there are at least three of them in a given list. This is accomplished by combining the capabilities of the
nth-last-child
pseudo-class and the general sibling combinator.
HTML
<h5>A list of four items (styled):</h5> <ol> <li>One</li> <li>Two</li> <li>Three</li> <li>Four</li> </ol> <h5>A list of two items (unstyled):</h5> <ol> <li>One</li> <li>Two</li> </ol>
CSS
/* If there are at least three list items, style them all */ li:nth-last-child(n + 3), li:nth-last-child(3) ~ li { color: red; }
Result
Specification |
---|
Selectors Level 4 # nth-last-child-pseudo |
BCD tables only load in the browser
with JavaScript enabled. Enable JavaScript to view data.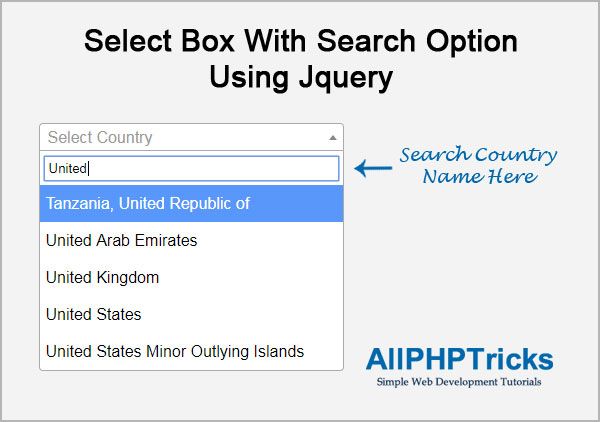
:nth-child
,:nth-last-of-type
- Quantity Queries for CSS
Last modified: , by MDN contributors
Pure JavaScript DOM Manipulation JavaScript DOM
Developers typically use jQuery when they need to do something with the DOM. However, almost any DOM manipulation can be done in pure JavaScript using its DOM API.
Consider this API in more detail:
-
- DOM queries
- How to work with lists?
- Adding classes and attributes
- Adding CSS styles
- Change DOM
- Methods for elements
- Event handlers
- Default action prevention
- Inheritance
- Animation
- Writing our library
- Application example
- Conclusion
At the end, you will write your own simple DOM library that can be used in any project.
DOM queries
This article introduces the fundamentals of the JavaScript DOM API. All the details and details are available on the Mozilla Developer Network. nine0003
DOM queries are made using the .querySelector()
method, which takes an arbitrary CSS selector as an argument.
const myElement = document.querySelector('#foo > div.bar')
It will return the first matching element. You can also check if an element matches a selector:
myElement.matches('div.bar') === true
If you want to get all elements that match a selector, use the following construct:
const myElements = document.querySelectorAll('.bar')
If you know which parent element to refer to, you can simply search through its child elements instead of searching all over the code:
const myChildElemet = myElement.querySelector('input[type="submit"]') // Instead of: // document.querySelector('#foo > div.bar input[type="submit"]')
So why use other, less convenient methods like .
? There is a small problem - the result of the output getElementsByTagName()
.querySelector()
is not updated, and when we add a new element (see section 5), it will not change.
const elements1 = document.querySelectorAll('div') const elements2 = document.getElementsByTagName('div') const newElement = document.createElement('div') document.body.appendChild(newElement) elements1.length === elements2.length // false
Also querySelectorAll()
collects everything in one list, which makes it not very efficient.
How to work with lists?
On top of that, .querySelectorAll()
has two little nuances. You can't just call methods on results and expect them to be applied to each of them (as you might be used to doing with jQuery). In any case, you will need to iterate over all the elements in the loop. Second, the returned object is a list of elements, not an array. Therefore, array methods won't work. Of course there are methods for lists too, something like .
, but, alas, they are not suitable for all cases. So it's better to convert the list to an array: forEach()
// Using Array.from() Array.from(myElements).forEach(doSomethingWithEachElement) // Or array prototype (pre-ES6) Array.prototype.forEach.call(myElements, doSomethingWithEachElement) // Simpler: [].forEach.call(myElements, doSomethingWithEachElement)
Each element has some properties that refer to a "family".
myElement.children myElement.firstElementChild myElement.lastElementChild myElement.previousElementSibling myElement.nextElementSibling
Because the element interface ( Element
) is inherited from the node interface ( Node
), the following properties are also present:
myElement.childNodes myElement.firstChild myElement.lastChild myElement.previousSibling myElement.nextSibling myElement.parentNode myElement.parentElement
The first properties refer to the element, while the last (except for .
) can be lists of elements of any type. Accordingly, you can check the type of the element: parentElement
myElement.firstChild.nodeType === 3 // this element will be a text node
Adding classes and attributes
Adding a new class is easy:
myElement.classList.add('foo') myElement.classList.remove('bar') myElement.classList.toggle('baz')
Adding a property to an element is the same as adding a property to any object:
// Get the value of an attribute const value = myElement.value // Set the attribute as a property of the element myElement.value = 'foo' // To set multiple properties, use .Object.assign() Object.assign(myElement, { value: 'foo', id: 'bar' }) // Removing an attribute myElement.value = null
Methods .getAttribute()
, .setAttribute()
, and .removeAttribute()
can be used. They will immediately change the element's HTML attributes (as opposed to DOM properties), which will trigger a browser redraw (you can see any changes by inspecting the element using the developer tools in the browser). Such redraws not only require more resources than setting DOM properties, but can also lead to unexpected errors.
Typically used for elements that don't have corresponding DOM properties, like colspan
. Or if their use is really necessary, for example for HTML-properties when inheriting (see Section 9).
Adding CSS styles
Adding them just like other properties:
myElement.style.marginLeft = '2em'
Some specific properties can be set using values after some calculations, it is better to use window.getComputedStyle()
. This method takes the element and returns a CSSStyleDeclaration containing the styles of both the element itself and its parent:0003
window.getComputedStyle(myElement).getPropertyValue('margin-left')
DOM change
Can move elements:
// Adding element1 as the last child of element2 element1.appendChild(element2) // Insert element2 as a child of element1 before element3 element1.insertBefore(element2, element3)
If you don't want to move, but need to insert a copy, use:
// Create a clone const myElementClone = myElement.cloneNode() myParentElement.appendChild(myElementClone)
The .cloneNode() method
takes a boolean value as an argument, if true
the child elements are also cloned.
Of course you can create new elements:
const myNewElement = document.createElement('div') const myNewTextNode = document.createTextNode('some text')
And then insert them as shown above. You can't remove an element directly, but you can do it through the parent element:
myParentElement.removeChild(myElement)
You can also refer indirectly:
myElement.parentNode.removeChild(myElement)
Methods for elements
Each element has properties such as .innerHTML
and .textContent
and, respectively, they contain HTML code , the text itself. The following example changes the element's content:
// Change the HTML myElement.innerHTML = `` // This is how the content is removed myElement.innerHTML = null // Append to HTML myElement.innerHTML += ` continue reading...New content
beep boop beep boop
nine0049Actually, changing the HTML is a bad idea, because it loses all the changes that were made earlier, and also overloads the event handlers. It is better to use this method only by completely discarding all HTML and replacing it with a copy from the server. Like this:
const link = document.createElement('a') const text = document.createTextNode('continue reading...') const hr = document.createElement('hr') link.href = 'foo.html' link.appendChild(text) myElement.appendChild(link) myElement.appendChild(hr) nine0049However, this will result in two browser redraws, while
.innerHTML
will only result in one.You can work around this by first adding everything to a DocumentFragment and then adding the fragment you need:
const fragment = document.createDocumentFragment() fragment.appendChild(text) fragment.appendChild(hr) myElement.appendChild(fragment)Event handlers
One of the simplest handlers:
myElement.onclick = function onclick (event) { console.log(event.type + 'got fired') } nine0049But generally it should be avoided. Here
.onclick
is a property of the element, and in theory you can change it, but you won't be able to add other handlers using another function that refers to the old one.To add event listeners, it's better to use
.addEventListener()
. It takes three arguments: an event type, a function that will be called whenever it fires, and a configuration object (we'll get to that later).myElement.addEventListener('click', function (event) { console.log(event.type + 'got fired') }) myElement.addEventListener('click', function (event) { console.log(event.type + 'got fired again') }) nine0049
Property
event.target
accesses the element to which the event is attached.And this way you can access all properties:
// The `forms` property is an array containing links to all forms const myForm = document.forms[0] const myInputElements = myForm.querySelectorAll('input') Array.from(myInputElements).forEach(el => { el.addEventListener('change', function (event) { console.log(event.target.value) }) }) nine0049Preventing default actions
To do this, use the
.preventDefault()
method, which blocks standard actions. For example, it will block form submission if client-side authorization was not successful:myForm.addEventListener('submit', function (event) { const name = this.querySelector('#name') if (name.value === 'Donald Duck') { alert('You gotta be kidding!') event.preventDefault() } }) nine0049The
.
helps if you have a specific event handler attached to the child element and a second handler for the same event attached to the parent.stopPropagation() method
As mentioned earlier, the
.addEventListener() method
takes an optional third argument as a configuration object. This object must contain any of the following boolean properties (by default, all set tofalse
):
- capture: The event will be attached to this element before any other element below in the DOM; nine0010
- once: An event can only be pinned once;
- passive:
event.preventDefault()
will be ignored (exception during error).
The most common property is .capture
, and it's so common that there's a shorthand for it: instead of passing it in a config object, just specify its value here:
myElement.addEventListener(type, listener, true )
Handlers are removed using the .removeEventListener() method, which takes two arguments: the event type and a reference to the handler to remove.
For example, property
once
can be implemented like this:
myElement.addEventListener('change', function listener (event) { console.log(event.type + ' got triggered on ' + this) this.removeEventListener('change', listener) })
Inheritance
Let's say you have an element and you want to add an event handler for all of its child elements. Then you would have to loop through them using method myForm.querySelectorAll('input')
as shown above. However, you can simply add elements to the form and check their content with event.target
.
myForm.addEventListener('change', function (event) { const target = event target if (target.matches('input')) { console.log(target.value) } })
And one more plus of this method is that the handler will be attached automatically to new child elements. nine0003
Animation
The easiest way to add animation is to use CSS with the property transition
. But for more flexibility (for example, for a game), JavaScript is better suited.
Calling the window.setTimeout() method Very smooth animation is achieved this way. In his article, Mark Brown discusses this topic. The fact that in the DOM, to perform any operations on elements all the time you have to iterate over them can seem quite tedious compared to jQuery syntax Now you have your own little library that contains everything you need. There are many more such helpers here. Now you know that you don't need to use a third-party framework to implement a simple modal or navigation menu. After all, the DOM API already has everything, but, of course, this technology has its drawbacks. The Basics of DOM Manipulation in Vanilla JavaScript (No jQuery) The Creative Bloq resource has published a material in which its authors shared with readers the best, in their opinion, examples of using JavaScript to create websites. The CPU chose the 30 most interesting resources. 229 102 views Using JavaScript, the authors of the article say, you can create memorable platforms that the user wants to return to. The language allows you to develop games, websites, APIs, and more. nine0003 Web designer Mike Kus' portfolio is "clean and understated," Creative Bloq editors write. It combines large images with simple user interface elements. “I think of my work as a brand. There is no need to add extra design elements to my site,” Kus says. Kus' portfolio is equally easy to navigate on all types of screens - he notes that this effect was the most difficult to achieve. Each of the designer's projects is presented with an image or photograph, so that the user wants to learn more about the work. nine0003 The website of the creative agency Hello Monday has undergone significant changes, the authors of the article note. The developers of the company have done a great job. They managed to make the interface user friendly. The site now contains examples of orders already completed by the agency - each project has its own page, which describes its history, which gives the user a deeper understanding of what Hello Monday is doing. "We were trying to get away from the stereotype of what a creative agency website should look like," says Cathy Hertel, head of the Hello Monday web page redesign project. The authors of the material find the site very attractive and responsive, which is facilitated by the organization of projects on the main page: it is automatically supplemented with new works of the agency when scrolling down. Multeor is an online multiplayer game written in JavaScript using the HTML5 canvas element. It was designed by Arjen de Vries and Philidor Weise and designed by Arthur van Hoog. The main task of the user in the game is to control the fall of meteorites, getting points for the destruction they left. The game uses a Node.js server to manage communication between desktop and mobile devices using WebSockets. Weise emphasizes that the development of Multeor did not use existing game libraries. nine0003 We wrote everything ourselves - it was very exciting, besides, we learned a lot of new things. The fact that we did not depend on specific assemblies and prefabs gave us a certain freedom of action: we did not have to deal with rendering existing graphics, handling collisions and separately describing the system responsible for explosions. Philidor Weise Crime Timelime is an aggregator that collects crime data in the UK using public APIs. “We organized the site so that it showed a map of the region and crime scenes for the month selected by the user in the panel below,” says project developer Alex Miller. The resource uses the Google Maps API, jQuery and jQRangeSlider were used to create the month panel. When the user interacts with the map, such as clicking on a specific location on the map, the site updates the image using JavaScript. The "bubbles" showing the number of crimes were created with CSS and animated with jQuery. nine0003 The Here is Today website uses JavaScript to create animations. The creator of the resource, designer Luke Twyman, explains his idea this way: “I wanted to create something that would give everyone a sense of the time scale. Here is Today helps to understand how vast the history of the universe is.” Twyman notes that he decided from the very beginning to abandon the standard measurements - pixels, and described his own, based on screen dimensions. This is the second JavaScript project created by the designer, and in it he applied elements that he did not use in the previous one. However, Twyman finds many similarities in the language with other high-level programming languages, besides, according to him, JavaScript has quite detailed documentation, and a large number of examples have been sorted out on the Internet (including on thematic forms). Tweetmap displays countries on the map in proportion to the number of tweets sent from them. The developer of the service, Rob Hawkes, lists several technologies used to create it: TopoJSON, D3.js, Node.js, PhantomJS, and special algorithms for building adjacent cartograms in real time. nine0003 We chose Node.js because we already had experience with it and because it's simple, fast, and flexible. To animate the map (in TopoJSON format), we use D3, a fantastic data visualization library. In particular, we make extensive use of the geo module, which allows us to do complex geographic calculations and transformations. To avoid problems with the presentation of maps in the client browser, the system generates maps on the server using D3, launches and renders them using the PhantomJS engine, and only then transfers them to the user - this avoids creating "holes" when displaying maps. nine0003 The Trip is an interactive movie created with JavaScript and HTML5 (no Flash). Otto Nascarella, the creator of the project, believes that the task of developing such a service turned out to be very difficult: Most of the difficulties we encountered were due to the fact that HTML5 at the time of writing the site did not have the means for cross-browser development. Then we decided that we would recommend customers to use Chrome. Otto Nascarella JavaScript site code uses jQuery for almost all tasks. The developers also used TextBlur and TextDrop to blur and animate text. This JavaScript page is the new portfolio and blog of design and marketing agency Si Digital. Crook used the jQuery.animate() method to achieve this effect. In addition, he adds, it was necessary to choose the right speed for filling the pipes with liquid - after all, everyone reads at different speeds. Interactive chart on the page with descriptions of completed projects and agency team, implemented using jQuery. The timeline, according to Crook, is dynamically generated based on information from the database using Ajax technology. Jean Halfstein is a web designer. In his portfolio, the authors of the article were most attracted by the main page - and the animation on it. “I had a really good time fiddling around with different effects. I really love using new technologies, so I decided to make the main page of my site a kind of sandbox - where I have fun with Three. The idea of the designer was to create a portfolio following modern trends - it had to be minimalistic, and at the same time bring something fresh to website design. So he decided to use mouse and drag controls to control animation rather than navigation keystrokes. When he designed the site, Jones said he had more experience with Flash than with JavaScript, but wanted to try something new to see what he could do. “I immediately realized that the syntax of JavaScript is almost the same as the syntax of ActionScript - so I quickly got the hang of it,” Jones describes the coding process. nine0003 Jones recalls that Flash did not give him access to work with mouse movements - it was a new experience that he really liked. The developer wanted to achieve a site responsiveness that he could not achieve using CSS class transitions. Jones is pleased with the result: If you're considering switching from ActionScript to JavaScript, don't hesitate. Nick Jones MapsTD is an online game, the essence of which is to defend the fortress. The fortress is the user's own home, which he must protect from the villains who tirelessly roam the streets of the area. Project creator Duncan Barclay explains how it works: “We used the Google Maps API, MooTools and JavaScript. The hardest part is finding the route that the user's enemies will follow. Once the player has chosen a starting point, the service searches for longitude and latitude, and calculates possible paths using Google. nine0003 As the game progresses, more and more opponents appear on the screen. Barclay says that developers had to "fight" with browser timing - the fact is that most of them reduce the frequency of checking for updates on the page over time, and it was necessary to make sure that this did not happen. Another problem was that as the game progressed, the number of enemies increased and performance decreased. As a result, the developers decided to increase the level of skills of the villains, and not their number. nine0003 The Glimpse resource was developed jointly by the Windows IE team and TheFind project, and combines TheFind search engine and the Facebook online shopping application of the same developers. As part of Glimpse, programmers have released their own framework based on Turn.js. The goal of the team from the very beginning was to make Glimpse a web application, not a regular website. The developers used the model-view-behavior paradigm, which separates the data model, user interface, and client interaction into three separate components. The service applies Thrift or JSON client-side model rendering templates, depending on the processing power of the client. nine0003 The Turn.js library was also used to develop catalogs. With the help of CSS and JavaScript, the models presented on the site are given volume - by applying shadows to the image. RBMA Radio uses the Modernizr tool, which enables cross-browser development with HTML5 and CSS. Modernizr is constantly updated, so site creators can improve the code as new features are introduced. In addition, when implementing the service, the Backbone.js library was used - with the help of it, tiles are loaded to create the effect of an endless page. nine0003 Nouvelle Vague is a site from the French design agency Ultranoir. The service allows you to "track" tweets for a given hashtag. It is implemented with JavaScript, WebGL and HTML5. HTML5, however, is only responsible for the teaser when opening the resource. One of the developers of the agency said that it took four months to create the site, and three people worked on it. “We were very interested in trying out WebGL,” he explains. The main goal of the project was to use 3D images to recreate the atmosphere from the video intro. The team has immersed itself in the new technologies of HTML5, CSS3 and JavaScript and believes these languages may become the standards for 3D work in the future due to their quality rendering, rich interoperability and responsiveness. The Convergence is a browser game with retro graphics reminiscent of Super Mario Bros. It showcases the amazing power of JavaScript and HTML5 and proves that HTML5 can do the job just as well as Flash. The developers promise to add audio and new levels to the game in the future, as well as support for the Mozilla Gamepad API. This web application makes the words "buy once, read on all devices" a reality. It uses HTML5 technologies, the JavaScript API, the jQuery and jQuery UI libraries, and several jQuery plugins, including jScrollPane for page scrolling and jQuery Templates. In addition, the development team has taken advantage of WebSQL for offline support. nine0003 The site launched by the WeFail studio, according to the authors of the note, looks scary, but still very cool. The resource is made in a rather sharp style. JavaScript is used to animate the interaction between the user and the system. To make scrolling only for individual interface elements, the team used the jQuery ScrollTo plugin. In addition, the Les Enfants code uses the jQuery Cycle plugin - it is responsible for displaying pictures and examples of the agency's work. nine0003 “We ran Les Enfants to see what performance options JavaScript has. It turned out that in combination with CSS Transform, you can achieve excellent results,” says developer Martin Hugh. Not too long ago, Hugh points out, you could only do things like this with Flash, but now it has a viable alternative: JavaScript. Pinterest is a prime example of using JavaScript to create an endless page effect. To create a site, jQuery tools, jQuery UI and the PageLess plugin were needed. nine0003 According to the authors of the note, PageLess is vital for Pinterest, because endless scrolling and loading of new Pins is much more effective in keeping the user's attention than social functions, such as commenting on posts. Love Bobm Builder helps users express their love or gratitude to someone. This is a neat and simple site that allows you to create and send a bombshell message. The resource uses the Modernizr tool to update JavaScript and HTML5 code in a timely manner. nine0003 When a user enters the site, the first thing he sees is the so-called "preloader" - it may seem that it is made using Flash, but it is not. HTML5 and JavaScript are responsible for filling the glass with beer as it loads. The resource uses partial scrolling - only for certain interface elements, and allows the user to interactively interact with images. By clicking on the animal masks, the client is sent to other scenes - all animation effects in which are performed using jQuery.animate (). nine0003 Trello is a collaborative or individual scheduling application where users can create lists of completed and outstanding tasks and share progress in real time. The site was developed using Node. Trello co-creator Daniel le Cerminand explains that using only one language when writing a site helps new team members to quickly integrate into the development process. Communication between users is implemented using Web Sockets - le Cherminand notes that this is a fairly new technology, so there were some difficulties when setting it up. nine0003 This retro-styled game by Little Workshop is designed to showcase the power of HTML5, JavaScript, and especially Web Sockets. It can support interaction between thousands of users at the same time. “Creating a multiplayer game is a great way to demonstrate how these technologies can work together. BrowserQuest relies on Node.js servers, each of which can run multiple instances of the game world, ”says studio developer Guillaume Lecolnay. nine0003 JS1k is an annual contest in which the task of participants is to create a JavaScript page on a given topic (most often animated images). The work of the 2012 winner Philip Buchanan originally weighed 8 KB - but in a day Philip was able to reduce its size to the required 1 KB by optimizing the tree generation algorithm. My tactic was to "honestly cheat" the compiler. For example, using the construction "a ? b : c" instead of "if (a) b else c" saves 8 bytes. nine0003 Philip Buchanan The service helps users create timelines and is very easy to use. You can include tweets, videos, photos, and audio recordings in an interactive timeline. You can describe your timeline using JSON or Google Docs - whichever is more convenient for the client. The main idea of the service is to provide the user with the opportunity to have some fun by taking part in an interactive story - helping a drawn person to overcome various obstacles. Sketches on the site are performed with the mouse. nine0003 jQuery and the vector graphics library Raphal. Panera Bread advertising website was created by Luxurios Animals agency. Studio CTO Dan Federman says that the developers decided from the very beginning to implement the project in CSS3 where possible, and use JavaScript for everything else. nine0003 CSS3 animation ensures smooth transitions on all types of devices. In addition, the team used the Hammer.js library for touch handling, Backbone.js for creating data models, the jQuery Transit and Isotope plugins, and HTML. Peanut Gallery is a project implemented by Google Creative Lab. The site allows users to add voiceovers to "silent" short films using a microphone. The resource uses the Google Speech API and JavaScript API - they help integrate speech recognition into web applications. nine0003 Walden Klump, one of the developers of the project, highlights in his opinion an interesting feature of the Google Speech API - dynamic speech recognition. Interactive Ear was created by Epiphany Solutions for Amphilon, a client who specializes in hearing aids and other solutions for the hearing impaired. The resource is aimed at children of primary and secondary school age. The site consists of three "showcases" showing how the human ear works. Project creator Brian James notes that by using JavaScript, HTML5, and CSS, the team was able to achieve peak performance even in outdated and unsupported browsers. Animations when examining the ear with a magnifier are GIFs. nine0003 Violin is a JavaScript code visualization tool. It shows how parts of an application interact with each other in real time.
until the animation is over is not a good idea, as your application may freeze, especially on mobile devices. It's better to use window.requestAnimationFrame() to save all changes until the next redraw. It takes a function as an argument, which in turn receives a timestamp:0003
const start = window.performance.now() const duration = 2000 window.requestAnimationFrame(function fadeIn (now)) { const progress = now - start myElement.style.opacity = progress / duration if (progress < duration) { window.requestAnimationFrame(fadeIn) } }
Writing our own library
$('.
. But why not write some of your own methods to make this task easier? foo').css({color: 'red'})
const $ = function $ (selector, context = document) { const elements = Array.from(context.querySelectorAll(selector)) return { elements, html(newHTML) { this.elements.forEach(element => { element.innerHTML = newHtml }) return this }, css(newCss) { this.elements.forEach(element => { Object.assign(element.style, newCss) }) return this }, on (event, handler, options) { this.elements.forEach(element => { element.addEventListener(event, handler, options) }) return this } } } nine0049
Usage example
Conclusion
For example, you always have to manually process lists of elements, while in jQuery this happens at the click of your fingers. nine0003
30 examples of great JavaScript sites — Offtop on vc.ru
nine0003
It allows users to find out how things are in their region. nine0003
This was done to make the site look the same on all devices. nine0003
Project Lead Alex Kruk explains the animation on the homepage this way: “Fluid moving through the pipes guides users through our portfolio - it activates image animations at each stage of the client’s exploration of the site.” nine0003
js and the HTML5 canvas element, ”says Halfstein. nine0003
While making my site, I wanted to understand if JavaScript is capable of what Flash is capable of. And I'm very impressed. nine0003
nine0003
To make the video appear not in pop-up windows, but directly on the site, the Shadowbox.js library was used.
js, MongoDB and Backbone.js.
Its weight should not exceed 1 KB.
js were used to create the game. Implementing the project with Raphal helped developers avoid performance issues on most devices and all browsers.
For example, if an English-speaking user begins to say "European Union", then the word "your" or "year" is recognized first, and only at the end it is converted to "European". Another useful feature is punctuation recognition. The phrases "question mark", "exclamation mark" and others automatically turn into their corresponding punctuation marks. nine0003